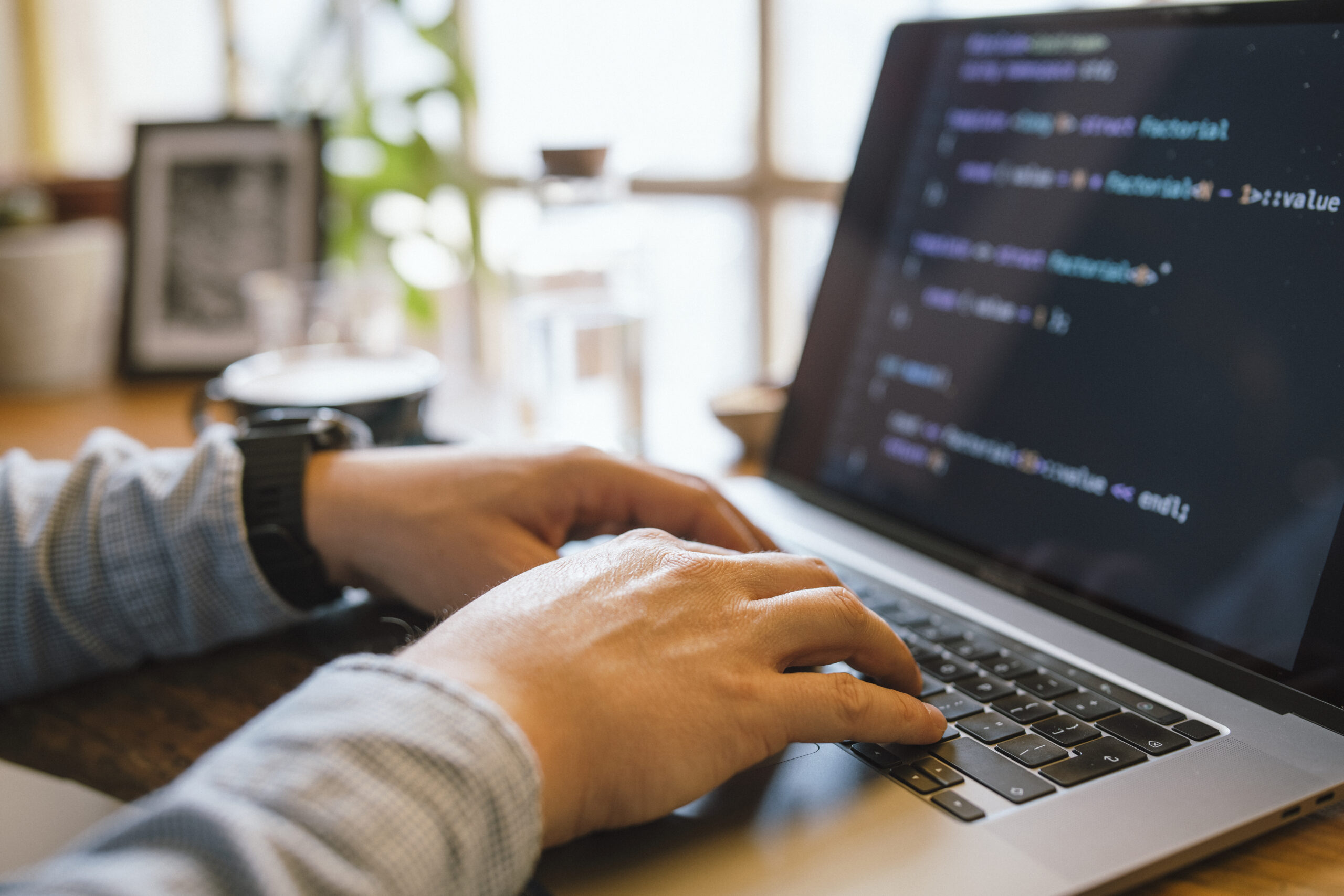
Debugging is one of the most important — nonetheless often ignored — abilities inside a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why issues go Incorrect, and Understanding to Feel methodically to resolve troubles proficiently. Whether you're a beginner or a seasoned developer, sharpening your debugging skills can save several hours of disappointment and drastically boost your productivity. Listed below are numerous techniques that can help developers degree up their debugging sport by me, Gustavo Woltmann.
Learn Your Applications
Among the list of fastest strategies developers can elevate their debugging abilities is by mastering the tools they use everyday. When crafting code is just one Section of advancement, understanding how to connect with it efficiently for the duration of execution is equally important. Modern-day enhancement environments come Geared up with potent debugging abilities — but a lot of developers only scratch the area of what these equipment can do.
Consider, for instance, an Built-in Progress Surroundings (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments assist you to established breakpoints, inspect the value of variables at runtime, step by code line by line, and also modify code on the fly. When utilised effectively, they Allow you to notice specifically how your code behaves all through execution, that's invaluable for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, keep track of network requests, watch actual-time performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can flip discouraging UI issues into manageable jobs.
For backend or system-degree builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep control in excess of functioning processes and memory management. Learning these resources could possibly have a steeper Discovering curve but pays off when debugging overall performance concerns, memory leaks, or segmentation faults.
Outside of your IDE or debugger, come to be relaxed with Model Command methods like Git to comprehend code heritage, come across the precise second bugs had been launched, and isolate problematic alterations.
Finally, mastering your applications means heading over and above default options and shortcuts — it’s about producing an personal expertise in your enhancement environment to ensure when troubles occur, you’re not missing in the dead of night. The higher you recognize your instruments, the greater time you could expend resolving the particular dilemma in lieu of fumbling by the procedure.
Reproduce the condition
One of the more significant — and infrequently neglected — methods in successful debugging is reproducing the situation. In advance of jumping in to the code or creating guesses, builders will need to make a reliable setting or situation exactly where the bug reliably seems. With no reproducibility, repairing a bug becomes a sport of chance, generally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is gathering just as much context as you possibly can. Ask issues like: What actions triggered The problem? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The more depth you've, the a lot easier it results in being to isolate the exact disorders beneath which the bug occurs.
As soon as you’ve collected ample data, try to recreate the situation in your local setting. This could indicate inputting exactly the same facts, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, contemplate crafting automated checks that replicate the edge situations or state transitions concerned. These assessments not only support expose the issue and also prevent regressions Later on.
From time to time, The difficulty could be natural environment-unique — it would materialize only on particular working devices, browsers, or less than specific configurations. Making use of tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the issue isn’t only a phase — it’s a attitude. It calls for tolerance, observation, and a methodical method. But after you can persistently recreate the bug, you happen to be already halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment a lot more properly, exam opportunity fixes properly, and connect more Evidently with all your workforce or buyers. It turns an abstract criticism right into a concrete obstacle — Which’s the place developers thrive.
Go through and Realize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Completely wrong. Rather then seeing them as frustrating interruptions, builders really should understand to treat mistake messages as immediate communications from your process. They typically let you know exactly what transpired, the place it occurred, and sometimes even why it transpired — if you know the way to interpret them.
Start off by reading through the message thoroughly and in full. Quite a few developers, especially when less than time force, glance at the first line and promptly commence making assumptions. But further inside the error stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into serps — go through and comprehend them to start with.
Split the mistake down into components. Can it be a syntax error, a runtime exception, or possibly a logic error? Does it stage to a certain file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the dependable code.
It’s also useful to be familiar with the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Discovering to recognize these can substantially speed up your debugging method.
Some glitches are imprecise or generic, and in Individuals conditions, it’s critical to look at the context in which the error transpired. Test related log entries, input values, and recent variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede larger concerns and provide hints about probable bugs.
Finally, error messages will not be your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When made use of effectively, it provides actual-time insights into how an application behaves, assisting you realize what’s taking place under the hood without needing to pause execution or step through the code line by line.
An excellent logging method begins with realizing what to log and at what level. Typical logging levels include DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for detailed diagnostic information during enhancement, Details for standard functions (like productive start off-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly once the method can’t go on.
Prevent flooding your logs with abnormal or irrelevant information. Too much logging can obscure significant messages and slow down your system. Concentrate on vital functions, state variations, input/output values, and important final decision points in the code.
Structure your log messages Plainly and regularly. Involve context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re In particular precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
In the long run, intelligent logging is about harmony and clarity. With a properly-assumed-out logging method, you may lessen the time it will take to spot difficulties, obtain further visibility into your applications, and improve the Total maintainability and dependability within your code.
Think Like a Detective
Debugging is not only a complex activity—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to approach the process like a detective resolving a secret. This mentality helps break down sophisticated troubles into workable sections and abide by clues logically to uncover the foundation result in.
Commence by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or effectiveness difficulties. Identical to a detective surveys a crime scene, gather as much related info as you are able to without having jumping to conclusions. Use logs, test cases, and user reviews to piece with each other a clear photograph of what’s going on.
Upcoming, sort hypotheses. Question by yourself: What could possibly be leading to this behavior? Have any adjustments not too long ago been produced to the codebase? Has this difficulty happened ahead of below comparable circumstances? The target should be to slender down prospects and determine potential culprits.
Then, exam your theories systematically. Try and recreate the trouble in a managed surroundings. In the event you suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally conceal in the minimum expected places—just like a lacking semicolon, an off-by-a single mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely understanding it. Momentary fixes might cover the real dilemma, just for it to resurface later.
And lastly, maintain notes on That which you tried and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future challenges and assist Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, solution issues methodically, and turn into more practical at uncovering concealed problems in sophisticated devices.
Write Tests
Composing assessments is one of the most effective strategies to transform your debugging competencies and General advancement effectiveness. Assessments not simply enable capture bugs early but will also serve as a safety Internet that provides you self confidence when building variations towards your codebase. A well-tested software is much easier to debug mainly because it lets you pinpoint particularly wherever and when a challenge takes place.
Begin with unit exams, which give attention to specific features or modules. These tiny, isolated exams can speedily reveal no matter whether a particular piece of logic is Operating as expected. Any time a exam fails, you promptly know the place to seem, substantially minimizing time invested debugging. Device checks are Specially valuable for catching regression bugs—concerns that reappear following Beforehand staying mounted.
Subsequent, combine integration tests and conclusion-to-conclude exams into your workflow. These help make sure a variety of elements of your software get the job done with each other smoothly. They’re specially valuable for catching bugs that arise in complicated units with a number of components or products and services interacting. If anything breaks, your tests can inform you which A part of the pipeline unsuccessful and below what disorders.
Composing exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you need to be aware of its inputs, expected outputs, and edge situations. This level of comprehension Normally leads to higher code composition and fewer bugs.
When debugging a concern, crafting a failing check that reproduces the bug is often a powerful initial step. As soon as the test fails persistently, you could center on correcting the bug and observe your take a look at go when the issue is settled. This tactic makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from the irritating guessing match right into a structured and predictable process—assisting you catch far more bugs, a lot quicker and more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to be immersed in the issue—observing your display screen for several hours, seeking solution following Remedy. But The most underrated debugging instruments is actually stepping absent. Getting breaks can help you reset your head, cut down irritation, and often see the issue from a new perspective.
If you're too near the code for much too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking noticeable faults or misreading code you wrote just several hours before. During this point out, your brain turns into significantly less effective at problem-resolving. A brief stroll, a coffee crack, or simply switching to another undertaking for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken time to disconnect, permitting their subconscious perform within the history.
Breaks also enable avert burnout, In particular for the duration of lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive and also draining. Stepping away allows you to return with renewed Electricity as well as a clearer state of mind. You may perhaps out of the blue observe a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
If you’re caught, a good guideline is to established a timer—debug actively for 45–60 minutes, then have a check here 5–ten moment split. Use that time to maneuver around, stretch, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging Over time.
Briefly, taking breaks is not really a sign of weak point—it’s a sensible strategy. It provides your Mind space to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is a lot more than just a temporary setback—It truly is a possibility to grow as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you something beneficial should you make the effort to replicate and review what went wrong.
Begin by asking oneself a number of essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught before with better procedures like unit testing, code evaluations, or logging? The answers usually reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've uncovered from a bug with your friends is usually In particular effective. Whether or not it’s via a Slack concept, a short generate-up, or a quick understanding-sharing session, encouraging Some others avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your way of thinking from stress to curiosity. Rather than dreading bugs, you’ll start out appreciating them as crucial aspects of your advancement journey. After all, many of the very best builders are not those who write best code, but those who continually master from their blunders.
Eventually, Each and every bug you deal with adds a completely new layer for your ability established. So subsequent time you squash a bug, have a instant to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Conclusion
Bettering your debugging techniques takes time, follow, and tolerance — however the payoff is big. It would make you a more successful, self-assured, and capable developer. The next time you are knee-deep in a very mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become far better at That which you do.